Structural directives-NgIf with HTML Elements
Here we will understand the use of NgIf with HTML elements. NgIf is an angular directive that is used to add a subtree of elements for the true value of expression. NgIf is used as * ngIf = "expression". Here if the value of "expression" is false or null, in both cases, the subtree of the element will not be added to the DOM.
NgIf can be used in different ways.
<div *ngIf="condition">...</div> <div template="ngIf condition">...</div> <ng-template [ngIf]="condition"><div>...</div></ng-template>
Now we provide an example of the NgIf directive.
Use the following code in app.component.ts file.
app.component.html
<h3>Use of NgIf</h3> <div> <input type="radio" name="radio1" (click)= "setValue(true)" checked > True <input type="radio" name="radio1" (click)= "setValue(false)"> False </div> <div *ngIf="isValid"> <b>Data is valid.</b> </div> <div *ngIf="!isValid"> <b>Data is invalid.</b> </div>
In the previous code, two </div> element uses NgIf. Based on the true and false condition, the respective </div> will be added or removed from the DOM. Use the following code in app.component.ts file.
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'app'; isValid: boolean = true; setValue(valid: boolean) { this.isValid = valid; } }
This will be the output of the above code.
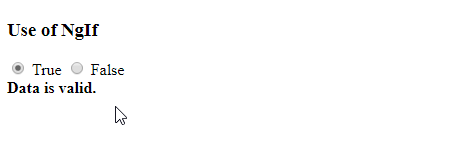