Improved Accessibility of Selects
The Angular team has also worked on enhancing the accessibility of the Selects in
the application for which they have used the native select element in the mat-form-field
.
The performance
, accessibility
, and usability
of the native select became better and efficient.
<mat-select>
is a form control for selecting a value from a set
of options, similar to the native <select> element. You can read in details
about selects in the Material Design spec. It is designed to work inside of an
<mat-form-field>
element.
To add options to the select, add <mat-option>
elements to the
<mat-select>
. Each <mat-option>
has a value
property that can be used to set the value which will be selected if the user chooses
this option. The content of the <mat-option>
is that which will
be shown to the user.
Note: To use a native select inside <mat-form-field>, add the matNativeControl attribute to the <select> element.
Example
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { MatButtonModule, MatMenuModule, MatToolbarModule, MatIconModule, MatCardModule, MatFormFieldModule, MatInputModule, MatDatepickerModule, MatDatepicker, MatNativeDateModule, MatRadioModule, MatSelectModule, MatOptionModule, MatSlideToggleModule } from '@angular/material'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, BrowserAnimationsModule, MatButtonModule, MatMenuModule, MatToolbarModule, MatIconModule, MatCardModule, BrowserAnimationsModule, MatFormFieldModule, MatInputModule, MatDatepickerModule, MatNativeDateModule, MatRadioModule, MatSelectModule, MatOptionModule, MatSlideToggleModule ], exports: [ BrowserAnimationsModule, MatButtonModule, MatMenuModule, MatToolbarModule, MatIconModule, MatCardModule, BrowserAnimationsModule, MatFormFieldModule, MatInputModule, MatDatepickerModule, MatNativeDateModule, MatRadioModule, MatSelectModule, MatOptionModule, MatSlideToggleModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { AngularVersions: Versions[] = [ { Id: '1', Value: 'Angular 2' }, { Id: '2', Value: 'Angular 4' }, { Id: '3', Value: 'Angular 5' }, { Id: '3', Value: 'Angular 6' }, { Id: '3', Value: 'Angular 7' } ]; } export interface Versions { Id: string; Value: string; }
<h4>Basic mat-select</h4> <mat-form-field> <mat-select placeholder="Angular Version"> <mat-option *ngFor="let angular of AngularVersions" [value]="angular.Id"> {{angular.Value}} </mat-option> </mat-select> </mat-form-field> <h4>Basic native select</h4> <mat-form-field> <select matNativeControl required> <option *ngFor="let angular of AngularVersions" [value]="angular.Id"> {{angular.Value}} </option> </select> </mat-form-field>
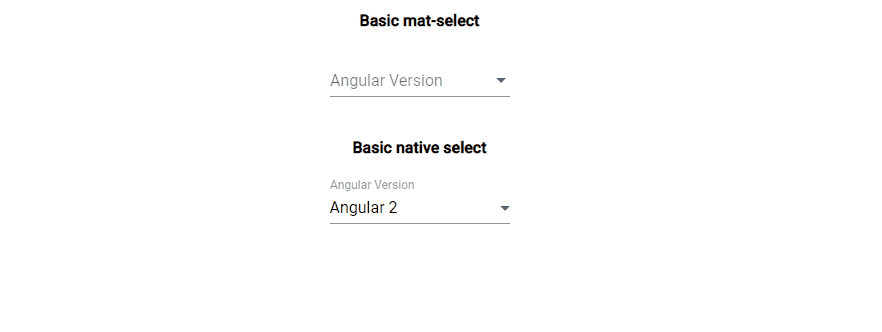
Note: To read in details go to material.angular.io